300x250
- 어떤 특정 데이터를 외부에 안보이게 하고 싶다면, 어떻게 해야할까? ex) 비밀번호라던지..
특정 JSON 비노출 처리하기
(1) @JsonIgnore 예제
@Data //getter,setter 사용
@AllArgsConstructor
public class User {
private Integer id;
@Size(min=2,message="Name은 두가지 이상 전달해 주세요") //최소 길이 2개 이상
private String name;
@Past //미래 데이터를 쓸수가 없다는 제약조건
private String joinDate;
@JsonIgnore
private String password;
@JsonIgnore
private String ssn;
}
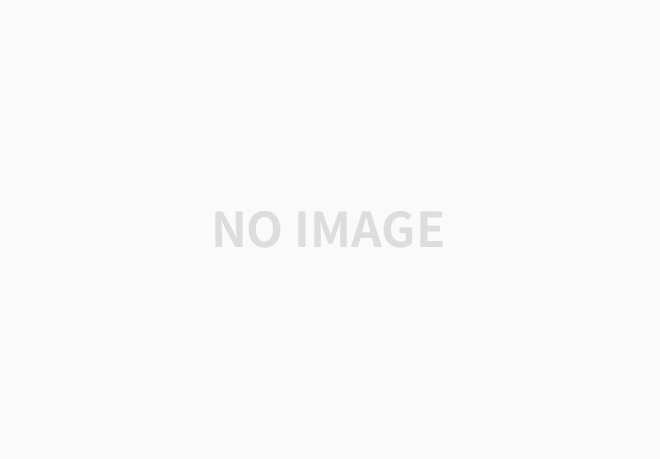
- password와, ssn 객체에 @JsonIgnore을 선언해본다.
- 결과로, 특정 도메인의 값이 노출 되지 않았다.
(2) @JsonIgnoreProperties 예제
package com.example.restfulwebservice.User;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import lombok.AllArgsConstructor;
import lombok.Data;
import javax.validation.constraints.Past;
import javax.validation.constraints.Size;
@Data
@AllArgsConstructor
@JsonIgnoreProperties(value = {"password"})
public class User {
private Integer id;
@Size(min=2,message="Name은 두가지 이상 전달해 주세요")
private String name;
@Past
private String joinDate;
private String password;
private String ssn;
}
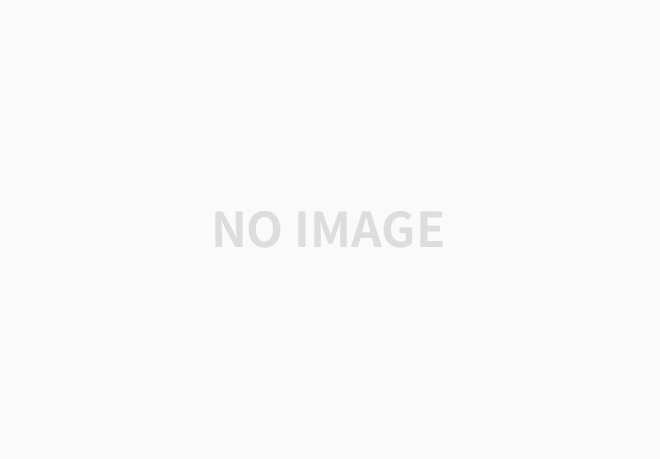
- @JsonIgnoreProperties에서 특정 프로퍼티 값을 배열 형태로 지정해주면 비노출 처리 가능하다.
(3) @JsonFilter 예제
@Data
@AllArgsConstructor
@JsonFilter("UserInfo")
public class User {
private Integer id;
@Size(min=2,message="Name은 두가지 이상 전달해 주세요") //최소 길이 2개 이상
private String name;
@Past
private String joinDate;
private String password;
private String ssn;
}
- 도메인 클래스에 @JsonFilter("필터명") 을 선언 해준다.
package com.example.restfulwebservice.User;
import com.fasterxml.jackson.databind.ser.FilterProvider;
import com.fasterxml.jackson.databind.ser.impl.SimpleBeanPropertyFilter;
import com.fasterxml.jackson.databind.ser.impl.SimpleFilterProvider;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.converter.json.MappingJacksonValue;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
@RequestMapping("/admin")
public class AdminUserController {
@Autowired
private UserDaoService service;
@GetMapping("/users/{id}")
public MappingJacksonValue retreiveAllUsers(@PathVariable int id) {
User user = service.findOne(id);
if(user == null){
throw new UserNotFoundException(String.format("ID[%s] not found",id));
}
SimpleBeanPropertyFilter filter = SimpleBeanPropertyFilter
.filterOutAllExcept("id","name","joinDate","ssn");
FilterProvider filters = new SimpleFilterProvider().addFilter("UserInfo",filter);
MappingJacksonValue mapping = new MappingJacksonValue(user);
mapping.setFilters(filters);
return mapping;
}
}
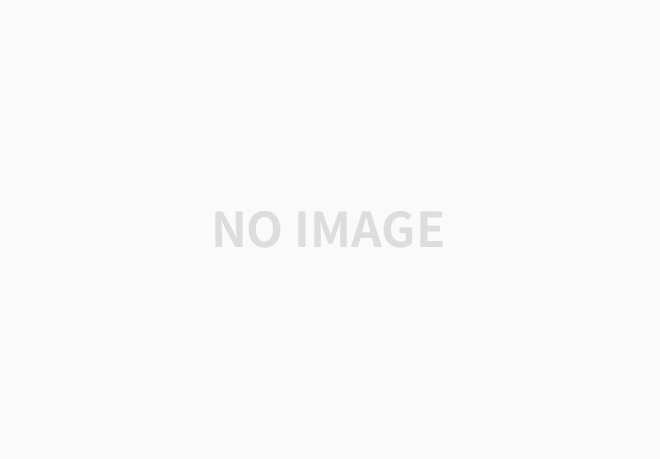
- Controller에서 Json을 필터링 할 수 있다.
- SimpleBeanPropertyFilter filter = SimpleBeanPropertyFilter.filterOutAllExcept()로 필터링할 프로퍼티들을 선언한다.
- FilterProvider를 사용해 Userinfo로 선언한 필터를 할당한다.
- Spring MVC MappingJackonValue를 사용해 user 객체와 filter 객체를 맵핑 후 리턴해준다.
- 필터로 지정해 준 값만 노출된다.
Reference
인프런 강의 - Spring Boot를 이용한 RESTful Web Services 개발
300x250
'공부 > Spring Boot를 이용한 RESTful Web Services 정리' 카테고리의 다른 글
[Spring Boot] RESTful Service 기능 확장 - 유효성 Validation API(@valid) (0) | 2021.08.19 |
---|---|
[Spring Boot] DELETE HTTP Method 구현 (0) | 2021.08.19 |
[Spring Boot] HTTP Status Code 제어, Exception Handling (0) | 2021.08.06 |
[Spring Boot] API 구현- Domain , GET , POST 예제 (0) | 2021.08.04 |
[Spring Boot] DispatcherServlet 작동 원리, @PathVariable 사용법 (0) | 2021.08.03 |